Did you know that every computer program you use, from your favorite app to the software running on your smartphone, is built upon a foundation called source code?
Source code is the bedrock of software development, containing the instructions and statements that define how a program functions.
Understanding source code is essential for programmers and developers, as it allows them to modify and improve program functions, build websites and web services, collaborate with other developers, execute programs, and facilitate learning through education.
In this article, we’ll explore the definition of source code and its strategic purposes in the world of programming and software development.
Key Takeaways:
- Source code is the fundamental component of a computer program that contains instructions and statements defining its behavior.
- Understanding source code allows programmers to modify and improve program functions according to specific requirements.
- Source code is essential for building websites, as it provides the foundation for web development.
- Developers can share their source code to foster knowledge sharing and collaboration within the programming community.
- Source code is translated into machine language and executed to perform specific program functions.
Table of Contents
What is a Source Code?
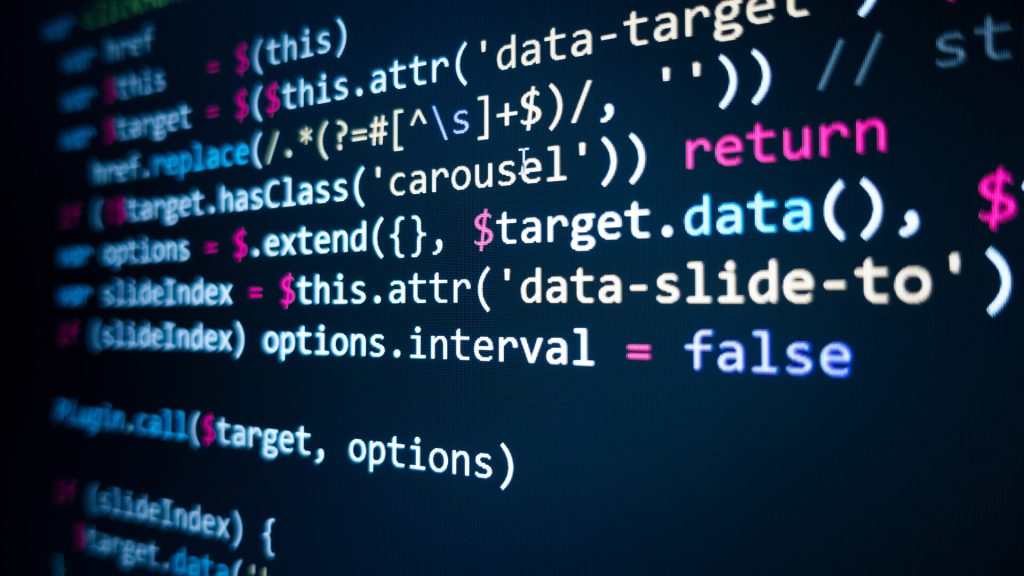
Source code refers to the collection of instructions and statements written by a programmer to create a computer program. It serves as the fundamental component of the program, defining its behavior and functionality. Source code is written using programming languages such as Python, Java, JavaScript, and more and is human-readable.
Programmers can understand and modify the source code to improve the functionality of existing programs or create new ones.
By analyzing the code, developers can identify bugs, optimize performance, add new features, and enhance user experience. Source code is the building block of software development, enabling innovation and advancement in technology.
5 Purposes of Source Code
Source code serves various strategic purposes in software development. These include improving program functions, building websites, sharing knowledge and collaborating, executing programs, and facilitating learning through education.
Purpose | Description |
---|---|
Improving Program Functions | Source code modification enhances program functionality by adding features, optimizing performance, and fixing bugs. |
Building a Website | Websites are created using source code written in HTML, CSS, and JavaScript, defining structure and functionality. |
Sharing Knowledge and Collaborating | Developers share source code to learn, contribute to projects, and collaborate, fostering community and skill growth. |
Executing Programs | Source code is translated into machine language for program execution, serving as an intermediary for human-readable code. |
Learning through Education | Source code provides a practical resource for learning programming techniques, languages, and real-world scenarios. |
1. Improving Program Functions
Modifying the source code allows programmers to improve the functionality of a program. By making changes to the code, they can add new features, optimize performance, fix bugs, and enhance the user experience. Understanding the source code is crucial for effectively modifying and improving program functions.
2. Building a Website
Source code plays a vital role in building websites. Web developers and programmers write the source code using programming languages such as HTML, CSS, and JavaScript to create the foundation and functionality of websites. The source code defines the structure, layout, and behavior of web pages, enabling developers to create interactive and user-friendly websites.
3. Sharing Knowledge and Collaborating
Source code can be shared among developers to foster knowledge sharing and collaboration. By sharing their source code, developers can learn from each other, contribute to open-source projects, and work together to solve programming challenges. It creates a sense of community and helps in the advancement of programming skills.
4. Executing Programs
The source code is translated into machine language through a process called compilation or interpretation. Once compiled, the resulting object code is executed to perform specific program functions. The source code serves as the intermediary between human-readable code and the machine-readable code required for program execution.
5. Learning through Education
Source code is an essential resource for learning programming and gaining a deeper understanding of how computer programs work. Students and aspiring programmers can study and analyze existing source code to learn programming techniques, best practices, and different programming languages. It provides practical insights into real-world computer programming scenarios.
How Does Source Code Work?
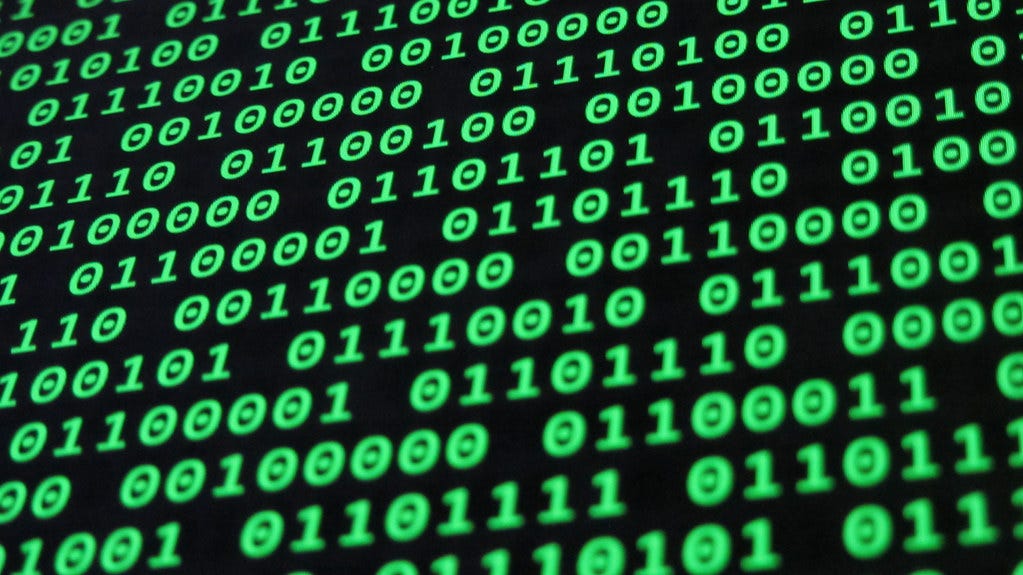
Source code is the backbone of programming logic and code execution. It functions by following a defined set of instructions and logic created by the programmer. These instructions are written in a programming language that serves as the intermediary between human-readable code and machine-executable code.
First, the programmer writes the source code using a specific programming language such as Python, JavaScript, or Java. The source code consists of a series of commands and statements that define the desired behavior of the program. It can include variables, functions, loops, conditionals, and more.
To convert the source code into an executable form, it needs to be translated into machine language that the computer can understand and execute. This translation is done by either an interpreter or a compiler, depending on the programming language and execution environment.
Interpreters read the source code line by line, executing each instruction as it encounters it. Interpreted languages like Python or JavaScript convert the source code into machine language on the fly, allowing for immediate execution. However, this process can be slower compared to compiled languages.
On the other hand, compilers translate the entire source code into machine language before execution. Compiled languages like C++ or Java generate an object code or executable file that contains the machine-language instructions. This resulting object code can be executed directly by the computer’s processor, resulting in faster and more efficient execution.
Once the source code is translated into machine language, the computer’s processor executes it, performing the desired functions outlined in the code. This can include anything from simple calculations to complex algorithms, depending on the program’s purpose.
Basic Structure of Source Code
Source code typically follows a structured format, comprising instructions written in a programming language. It begins with declarations and imports, defining variables and libraries needed for the program.
The main body contains the program’s logic, including functions, loops, and conditional statements, organized in a readable and logical manner. Comments may be interspersed to explain code sections or provide context.
Finally, the source code concludes with closing statements or cleanup routines.
Examples of Source Code in Various Programming Languages
Programming Language | Example Code |
---|---|
Python | def greet(name): print("Hello, " + name + "!") greet("John") |
JavaScript | function greet(name) { console.log("Hello, " + name + "!"); } greet("John"); |
Java | public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } } |
C++ | #include <iostream> int main() { std::cout |
HTML | <!DOCTYPE html> <html> <head> <title>Hello, World!</title> </head> <body> <h1>Hello, World!</h1> </body> </html> |
CSS | h1 { color: blue; font-size: 24px; } p { color: red; font-size: 16px; } |
Ruby | def greet(name) puts "Hello, #{name}!" end greet("John") |
PHP | <?php $name = "John"; echo "Hello, " . $name . "!"; ?> |
Swift | import UIKit func greet(name: String) { print("Hello, \(name)!") } greet(name: "John") |
SQL | SELECT * FROM customers WHERE country = 'USA'; |
1. Python
Python is a widely used programming language known for its simplicity and readability. Below is an example of Python source code:
def greet(name):
print("Hello, " + name + "!")
greet("John")
2. JavaScript
JavaScript is a programming language used for client-side scripting in web development. Below is an example of JavaScript source code:
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("John");
3. Java
Java is an object-oriented programming language commonly used for enterprise-level applications. Below is an example of Java source code:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
4. C++
C++ is a powerful programming language used for systems programming and performance-critical applications. Below is an example of C++ source code:
#include
int main() {
std::cout
5. HTML
HTML is a markup language used for structuring web pages. Below is an example of HTML source code:
<!DOCTYPE html>
<html>
<head>
<title>Hello, World!</title>
</head>
<body>
<h1>Hello, World!</h1>
</body>
</html>
6. CSS
CSS is a style sheet language used for styling web pages. Below is an example of CSS source code:
h1 {
color: blue;
font-size: 24px;
}
p {
color: red;
font-size: 16px;
}
7. Ruby
Ruby is a dynamic scripting language known for its simplicity and elegance. Below is an example of Ruby source code:
def greet(name)
puts "Hello, #{name}!"
end
greet("John")
8. PHP
PHP is a server-side scripting language used for web development. Below is an example of PHP source code:
<?php
$name = "John";
echo "Hello, " . $name . "!";
?>
9. Swift
Swift is a programming language developed by Apple for iOS app development. Below is an example of Swift source code example:
import UIKit
func greet(name: String) {
print("Hello, \(name)!")
}
greet(name: "John")
10. SQL
SQL is a language used for managing and querying databases. Below is an example of SQL source code:
SELECT * FROM customers WHERE country = 'USA';
Programming Language Example Code Python
def greet(name):
print("Hello, " + name + "!")
greet("John")
6 Types of Source Code
Source code can be classified into different types based on its characteristics and usage. This section explores six main types of source code: interpreted source code, compiled source code, software feature source code, open-source code, closed-source code, and version-controlled source code.
Type | Description |
---|---|
Interpreted Source Code | Source code executed directly by an interpreter without prior compilation, common in scripting languages like Python or JavaScript. Allows for quick execution but may be slower than compiled code. |
Compiled Source Code | Source code transformed into machine code through compilation, resulting in faster and more efficient execution than interpreted code. |
Software Feature Source Code | Source code adding specific features or functionalities to a program, enhancing its capabilities through custom code. |
Open-Source Code | Publicly accessible code that can be freely used, modified, and distributed, fostering collaboration and community involvement. |
Closed-Source Code | Proprietary code not publicly accessible, typically associated with closed-source software to protect intellectual property. |
Version-Controlled Source Code | Source code managed using version control systems, facilitating tracking of modifications, collaboration, and code integrity. |
1. Interpreted Source Code
Interpreted source code is executed directly by an interpreter without the need for prior compilation. It is commonly used in scripting languages such as Python, JavaScript, and Perl. Interpreted source code allows for easy modification and quick execution, but it may be slower compared to compiled code.
2. Compiled Source Code
Compiled source code is transformed into machine code through a compilation process. It is converted into a binary format that can be directly executed by the computer’s processor. Compiled source code typically results in faster and more efficient execution compared to interpreted code.
3. Software Feature Source Code
Software feature source code refers to the source code that adds specific features or functionalities to a software program. It allows developers to enhance the capabilities of a program by writing custom code for additional features. Software feature source code follows the structure and conventions of the existing program.
4. Open-Source Code
Open-source code is publicly accessible and can be freely used, modified, and distributed by anyone. It promotes collaboration and community involvement by allowing developers to contribute to the codebase and improve the software collectively. Open-source code is often governed by licenses that define the terms of use and distribution.
5. Closed-Source Code
Closed-source code refers to source code that is not publicly accessible. It is typically associated with proprietary software developed by companies. The source code is kept private to protect intellectual property and maintain control over the software. Closed-source code is distributed in compiled form, and modifications can only be made by the original or only the software authors themselves.
6. Version-Controlled Source Code
Version-controlled source code is managed using source code management systems, also known as version control systems. These systems track modifications to the source code, maintain a complete history of changes, and enable collaboration among developers. Version-controlled source code allows for easy tracking of code changes, resolving conflicts, and maintaining code integrity.
Source Code vs. Object Code vs. Executable Code
In the process of creating and running a computer program, there are three distinct stages: source code, object code, and executable code. Each stage plays a vital role in program development and execution.
Let’s explore the differences between these stages and understand their significance:
Source code is the human-readable form of a program that is written by programmers using programming languages like Python, Java, or C++. It consists of instructions and statements that define the program’s behavior. Programmers can modify the program’s source code to add features, fix bugs, or optimize performance.
Object code, which consists of binary code,is the result of translating the source code into machine-readable instructions. This translation is achieved by using a compiler or an assembler. Object code is not directly executable by the computer; it contains binary instructions that represent the source code in a format that the computer understands.
Executable code is the final stage of program development. It is the machine code that can be directly executed by the computer’s processor. Executable code is created by linking the object code with any necessary libraries or dependencies. The executable file is what users run to execute the program and perform the desired functions.
To summarize:
- Source code is the human-readable form of a program written by programmers.
- Object code is the machine-readable version of the source code.
- Executable code is the final stage where the program is ready to be executed by the computer.
Stage | Description |
---|---|
Source Code | The human-readable form of the program written by programmers. |
Object Code | The machine-readable version of the source code. |
Executable Code | The final stage where the program is ready to be executed by the computer. |
Licensing of Source Code and Why it Is Important
Source code licensing is a critical aspect of software development that governs the proper use, modification, and distribution of source code. By establishing legal terms and conditions, licensing ensures the protection of intellectual property rights and defines the rights and responsibilities of users. It also plays a significant role in ensuring compliance with legal requirements.
There are different types of open-source software and code licensing, including open-source licensing and proprietary licensing. Open-source licensing allows for the free use, modification, and distribution of source code, promoting collaboration and community involvement. Proprietary licensing, on the other hand, restricts access to the source code and is commonly used for proprietary software, protecting intellectual property rights and maintaining control over the software.
Intellectual property protection is a crucial aspect of source code licensing. By implementing the right licensing agreements, software developers can safeguard their intellectual property and prevent unauthorized use or distribution of their source code. This protection is essential for commercial software products and innovative software solutions.
Additionally, licensing provides clarity and legal certainty for both software developers and users. It outlines the rights and obligations of each party and establishes clear guidelines for the permissible uses of the source code. This helps avoid legal disputes and ensures that software development is conducted legally.
7 Benefits of Understanding Source Code
Understanding source code offers several benefits to programmers and software developers. This section highlights seven key benefits, including the ability to debug and troubleshoot code, customize and modify software, learn and develop programming skills, collaborate with teammates, perform security assessments, optimize performance, and ensure compliance with regulations.
Benefit | Description |
---|---|
Debugging and Troubleshooting | Understanding source code aids in debugging and troubleshooting, helping developers locate and fix bugs for reliable software. |
Customization and Modification | Knowledge of source code enables developers to customize and modify software to meet specific needs, enhancing its value. |
Learning and Skill Development | Studying source code facilitates learning programming techniques and developing coding skills across various languages. |
Collaboration and Teamwork | Source code comprehension fosters collaboration among developers, allowing them to work together on projects effectively. |
Security and Vulnerability Assessment | Understanding source code helps developers assess software security, identify vulnerabilities, and implement safeguards. |
Performance Optimization | Source code analysis assists in optimizing software performance by identifying and addressing performance bottlenecks. |
Compliance and Regulation | Knowledge of source code ensures compliance with regulations, enabling developers to protect user data and meet legal standards. |
1. Debugging and Troubleshooting
Understanding source code allows developers to effectively debug and troubleshoot software. By analyzing the code, identifying errors, and tracing the execution flow, programmers can locate and fix bugs, improving the overall, code quality and reliability of the program.
2. Customization and Modification
With knowledge of source code, developers can customize and modify software to meet specific requirements and provide tailor-made solutions. They can add new features, customize user interfaces, and optimize functionality based on user needs, enhancing the usability and value of the software.
3. Learning and Skill Development
Studying and understanding source code is an effective way to learn programming and develop programming skills. By examining well-written code, aspiring programmers can learn coding techniques, best practices, and gain insights into different programming languages operating systems, and frameworks.
4. Collaboration and Teamwork
Source code comprehension facilitates collaboration and teamwork among developers. It enables team members to understand and contribute to a shared codebase, collaborate on projects, review and provide feedback on code, and work together to create high-quality software.
5. Security and Vulnerability Assessment
Understanding source code enables developers to assess the security of their software and identify potential vulnerabilities. By conducting code reviews, analyzing potential security risks, and implementing secure coding practices, they can enhance the security posture of the program and protect against potential threats and attacks.
6. Performance Optimization
Source code comprehension allows developers to optimize the performance of software by identifying and addressing performance bottlenecks. By analyzing the code, removing unnecessary operations, and optimizing algorithms, programmers can enhance the efficiency and speed of the program, providing a better user experience.
7. Compliance and Regulation
Understanding source code is crucial for ensuring compliance with legal requirements and regulations. By reviewing the source code files, developers can identify potential compliance issues, implement security measures, protect user data, and adhere to industry-specific regulations, such as data privacy laws and security standards.
Conclusion
In conclusion, source code is the foundation of computer programming language and software development. Programmers write source code because it serves as the blueprint that defines how a computer program functions, allowing developers to customize and optimize software to meet specific needs.
Understanding source code is essential for programmers to enhance their skills and create innovative software. By delving into the world of source code, developers can learn different programming languages, gain insights into best practices, and contribute to the development community.
Moreover, source code plays a crucial role in collaboration and teamwork. By sharing and reviewing source code, developers can work together to solve complex problems and create high-quality software.
The ability to analyze and troubleshoot source code also enables programmers to debug errors, fix bugs, and improve the overall reliability of their programs.
Author’s Bio:
Scott McAuley is the CEO of TMG Voice. Great teamwork starts with TMG Voice, where all your people, tools, and communication come together for faster and more flexible work.
We offer unparalleled phone service with EPIC support from real humans, no long-term contracts, and the lowest prices with the greatest features. Experience seamless, cost-effective, and personalized telecommunications solutions tailored to your business’s unique needs.
For more information about TMG Voice’s Business Communication services, visit our Plans and Pricing page or contact us using our hotline (832) 862-6900. You can also visit our office at 21175 Tomball Parkway #361 Houston, Texas 77070, or send a message to our email through our Contact Us page.
Discover Strategic Purposes of Source Code for Business
Want to understand the role of source code in development? Learn more in our blogs and explore TMGVoice for tools that boost your coding efforts.
Empower your projects today!
FAQ
What Does Source Code Mean?
Source code refers to the human-readable instructions written in a programming language that developers use to create software applications.
What is Source Code in HTML?
In HTML, source code refers to the markup language used to create web pages. It consists of HTML elements, tags, attributes, and content that define the structure and layout of web documents.
What is an Integrated Development Environment (IDE)?
An integrated development environment (IDE) is a software application that provides comprehensive tools and features to facilitate software development, including code editing, debugging, compiling, and project management capabilities in a single integrated interface.
How do you Identify Source Code?
Source code can be identified by its human-readable format written in a programming language, typically containing keywords, syntax, and structures specific to the chosen language, such as Java, Python, or C++.